Individual Project Final
Value of the Timebox
- Keep track of what projects were done at certain weeks
- Library of past lessons and code
- Helped facilitate weekly gols and progress in projects
Contributions to Project
Frontend Cover / CSS Page
- FIFA inspired colors
- HTML attribute buttons, open a pack page, linking buttons to different sections of the frontend
AWS Deployment
- Walked through deployment until certbot config
Problems
- API and backend not setup properly, led to improper AWS setup
Solutions
- Learned about API setup before trying the deployment process again (With Aidan)
- Aidan and I walked through each step together, deployed the backend successfully
Commits/Analytics
- Multiple merge errors encountered, worked out after sorting things out with merge editor
API
- (Tried but failed) Created
Previous API attempt / mistakes
- No API model
- Improper endpoints on frontend and backend
- Over reliance on AI help for checking code
from flask import Flask, jsonify
from flask_cors import CORS # Import CORS module
app = Flask(__name__)
CORS(app) # Enable CORS for your app
# Define position values
CF = "Center Forward"
## PLAYER POSITIONS
players = [
(Player("Lionel Messi", CF, 90, 80, 87, 90, 94, 33, 64), 1 / 100),
(Player("Cristiano Ronaldo", ST, 86, 85, 90, 75, 92, 45, 78), 1 / 86),
(Player("Erling Haaland", ST, 91, 89, 93, 66, 80, 24, 88), 1 / 91),
## PLAYER LIST
@app.route('/get_random_player', methods=["GET"])
def get_random_player():
random_player, _ = random.choices(players, weights=weights)[0]
player_info = {
"Name": random_player.name,
"Position": random_player.pos,
"Overall": random_player.ovr,
"Pace": random_player.pac,
"Shooting": random_player.sho,
"Passing": random_player.pas,
"Dribbling": random_player.dri,
"Defense": random_player.defe,
"Physicality": random_player.phy
}
return jsonify(player_info)
if __name__ == '__main__':
app.run()
Team Communication
- Pushed to have team start on project and helped split workload on which parts needed more work
Reflection / What I would improve
- Better communication with team in order to keep each other in check and on task
- Seek out more help from peers and teachers
- API: Look into proper creation based on jokes.py and understand API models and fetch functions
- Figure out the error that we had last friday with “players”
My Favorite Types of Code / Most Understood Code
Python
- if, else, elif functions (Python Quiz)
HTML
- Head, Body, and
attributes
Treasure Hunt: Python
print("Welcome to my treasure hunt, answer all the questions right to receive the treasure! Let's begin")
def ask_question(question, correct_answers):
while True:
player_answer = input(question + " ").lower()
if player_answer in correct_answers:
print(player_answer)
print("Correct! Here's the next question...")
return True
else:
print(player_answer)
print("WRONG ANSWER. But let's continue anyways...")
return False
questions = 3
correct = 0
question1 = "What gets wet when drying?"
answer1 = ["towel", "a towel"]
question2 = "What has a head, a tail, but no body?"
answer2 = ["coin", "a coin"]
question3 = "This is as light as a feather, yet no one can hold it, what is it?"
answer3 = ["your breath", "breath"]
if ask_question(question1, answer1):
correct += 1
if ask_question(question2, answer2):
correct += 1
if ask_question(question3, answer3):
correct += 1
print("Congratulations! You've completed the treasure hunt!")
score = correct
print("You scored " + str(score) + " / 3")
if score == 3:
print("You get the big prize: https://upload.wikimedia.org/wikipedia/commons/thumb/7/7b/Obverse_of_the_series_2009_%24100_Federal_Reserve_Note.jpg/640px-Obverse_of_the_series_2009_%24100_Federal_Reserve_Note.jpg")
elif score == 2:
print("You get the medium prize https://upload.wikimedia.org/wikipedia/commons/thumb/0/09/50_USD_Series_2004_Note_Front.jpg/640px-50_USD_Series_2004_Note_Front.jpg")
else:
print("You did not score any correct answers, so you get no prize.")
Welcome to my treasure hunt, answer all the questions right to receive the treasure! Let's begin
towel
Correct! Here's the next question...
coin
Correct! Here's the next question...
fhgh
WRONG ANSWER. But let's continue anyways...
Congratulations! You've completed the treasure hunt!
You scored 2 / 3
You get the medium prize https://upload.wikimedia.org/wikipedia/commons/thumb/0/09/50_USD_Series_2004_Note_Front.jpg/640px-50_USD_Series_2004_Note_Front.jpg
%%html
<head>
<p style="color: red"><strong> MOVIE GALLERY 2023</strong></p>
<body>
<div><img src="https://m.media-amazon.com/images/M/MV5BYzIxOTk1NDQtMzJlOC00ODZlLWE1YTAtNTA5ODZlZmZmMDBhXkEyXkFqcGdeQXVyMjkwOTAyMDU@._V1_FMjpg_UX1000_.jpg" alt="Creed 3" width=200 height=200> </div>
<div><img src="https://bethelbearfacts.com/wp-content/uploads/2023/05/guardians-of-the-galaxy-vol-three-newbutton-1676306275720.jpeg" alt="GOTG 3" width=200 height=200> </div>
<a href="https://m.media-amazon.com/images/M/MV5BNTIzZjMyOGQtZTQ4OS00MmJhLTg0MmEtM2YwMWU0YWFjNzU3XkEyXkFqcGdeQXVyMTY3ODkyNDkz._V1_.jpg">
<button id="Button">Equalizer 3</button>
</body>
MOVIE GALLERY 2023

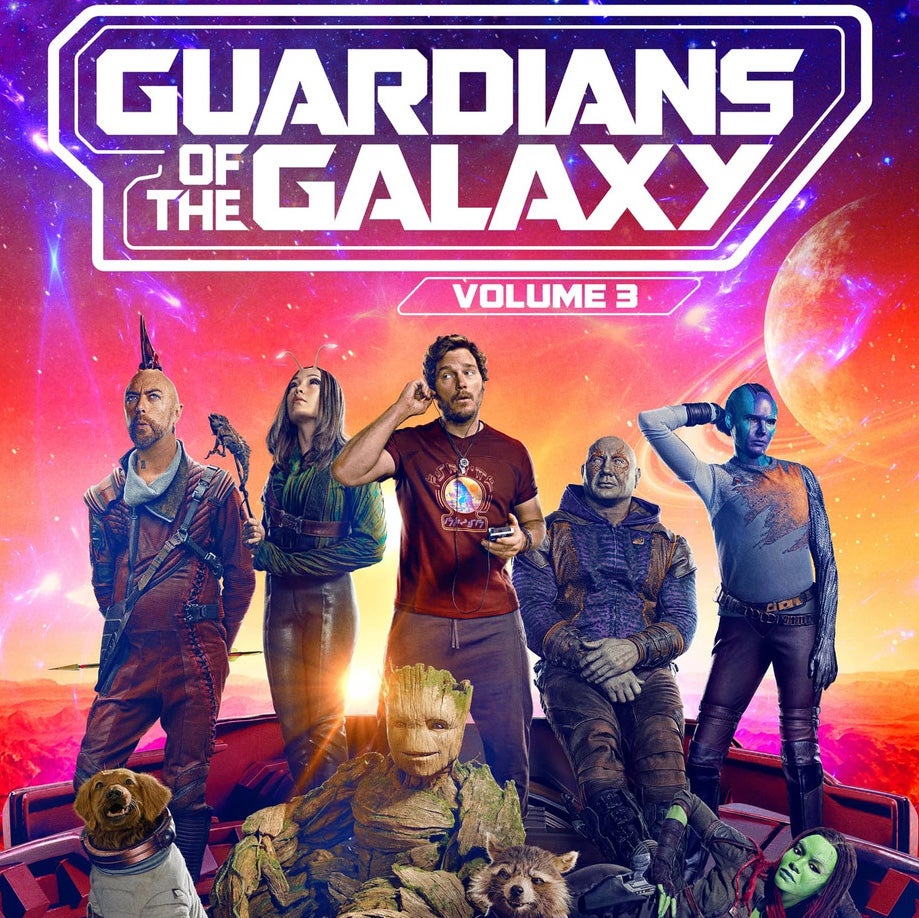